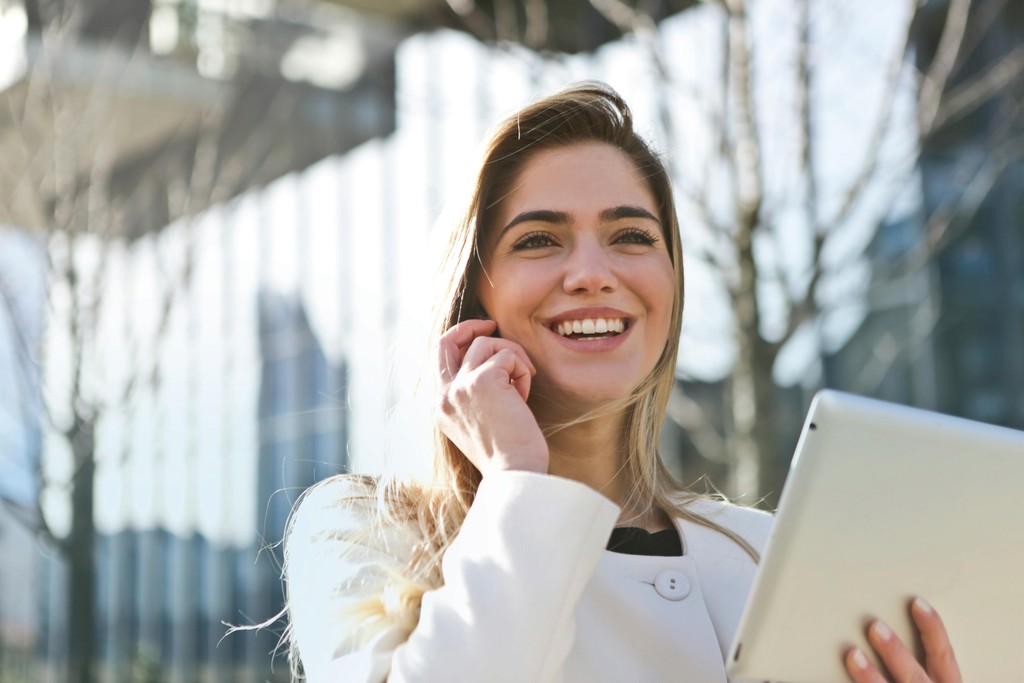
Blog /
Blog /
30 Most Common Exception Handling Interview Questions You Should Prepare For
30 Most Common Exception Handling Interview Questions You Should Prepare For
30 Most Common Exception Handling Interview Questions You Should Prepare For
Mar 5, 2025
Mar 5, 2025
30 Most Common Exception Handling Interview Questions You Should Prepare For
Written by
Written by
Jason Bannis
Jason Bannis
Introduction to Exception Handling Interview Questions
Preparing for interviews, especially those involving technical topics like exception handling, can be daunting. However, mastering common interview questions can significantly boost your confidence and performance. This guide covers 30 of the most frequently asked exception handling interview questions, providing you with insights, strategies, and sample answers to help you ace your next interview.
What are Exception Handling Interview Questions?
Exception handling interview questions are designed to evaluate your understanding of how to manage errors and unexpected events in a program. These questions assess your knowledge of exception types, handling mechanisms, and best practices for writing robust and reliable code. Interviewers use these questions to gauge your ability to prevent application crashes, ensure graceful error recovery, and maintain a positive user experience even when things go wrong.
Why Do Interviewers Ask Exception Handling Questions?
Interviewers ask exception handling questions to determine if you can write stable, maintainable, and user-friendly applications. They want to see if you understand:
Error Management: How well you can anticipate and handle potential errors.
Code Robustness: Your ability to write code that doesn't crash unexpectedly.
Debugging Skills: How effectively you can identify and fix issues.
Best Practices: Whether you follow recommended guidelines for exception handling.
Problem-Solving: Your approach to resolving unexpected issues in a systematic way.
By evaluating these aspects, interviewers can assess whether you have the skills necessary to build resilient software that can withstand real-world conditions.
Preview of 30 Exception Handling Interview Questions
What is an Exception in Java?
What is the Superclass of All Exceptions in Java?
What are the Types of Exceptions in Java?
How to Handle Checked Exceptions?
What is the Difference Between Checked and Unchecked Exceptions?
How to Catch Multiple Exceptions?
What is the Use of Finally Block?
What is Exception Handling?
Explain the try-catch block.
What is the purpose of the throws keyword?
What are custom exceptions?
How do you create a custom exception in Java?
What is the difference between throw and throws?
Can a finally block be skipped?
What happens if an exception is not caught?
Explain exception propagation.
What is the difference between Error and Exception?
Can you have an empty catch block? Is it good practice?
How does exception handling affect performance?
What are some best practices for exception handling?
How do you log exceptions?
What is the purpose of chained exceptions?
How do you re-throw an exception?
What is try-with-resources in Java?
Can you use multiple try-catch blocks?
What are some common exceptions in Java?
How do you handle exceptions in multi-threaded environments?
Explain the concept of exception masking.
What are the advantages of using exceptions?
How do you design an exception handling strategy for a large application?
30 Exception Handling Interview Questions
1. What is an Exception in Java?
Why you might get asked this:
Interviewers ask this question to gauge your basic understanding of what exceptions are and their role in program execution. It helps determine if you can define the concept accurately.
How to answer:
Define an exception as an event that disrupts the normal flow of the program.
Explain that exceptions occur during runtime due to various factors.
Mention examples such as network errors, database connection issues, or logical errors.
Example answer:
"An exception in Java is an event that occurs during the execution of a program, disrupting its normal flow. It can be caused by various factors such as network errors, database connection issues, or logical errors in the code."
2. What is the Superclass of All Exceptions in Java?
Why you might get asked this:
This question tests your knowledge of the Java exception hierarchy and the root class from which all exceptions are derived.
How to answer:
Identify
Throwable
as the superclass for all exceptions and errors.Explain that
Exception
is a subclass ofThrowable
and serves as the superclass for most exceptions.Mention that
Error
is another subclass ofThrowable
, representing serious problems that are generally unrecoverable.
Example answer:
"The superclass of all exceptions in Java is Throwable
. Exception
is a subclass of Throwable
and serves as the superclass for most exceptions that a program might want to catch. Error
is another subclass of Throwable
, representing serious problems like OutOfMemoryError
that are generally unrecoverable."
3. What are the Types of Exceptions in Java?
Why you might get asked this:
This question assesses your understanding of the different categories of exceptions in Java and how they are classified.
How to answer:
Describe the two main types: checked and unchecked exceptions.
Explain that checked exceptions are anticipated and must be handled at compile time.
Explain that unchecked exceptions occur due to programming errors and do not require explicit handling.
Mention custom exceptions as user-defined exceptions.
Example answer:
"There are two main types of exceptions in Java: checked and unchecked. Checked exceptions, like IOException
, must be handled at compile time, typically using a try-catch block or a throws clause. Unchecked exceptions, like NullPointerException
, occur due to programming errors and do not require explicit handling. Additionally, there are custom exceptions, which are user-defined exceptions created by extending the Exception
class."
4. How to Handle Checked Exceptions?
Why you might get asked this:
This question evaluates your practical knowledge of how to deal with exceptions that the compiler forces you to handle.
How to answer:
Explain that checked exceptions can be handled using a try-catch block.
Describe the alternative of declaring the exception with a throws clause in the method signature.
Highlight the importance of handling or declaring checked exceptions to avoid compilation errors.
Example answer:
"Checked exceptions can be handled in two primary ways: using a try-catch block to catch and handle the exception, or by declaring the exception with a throws clause in the method signature, which passes the responsibility of handling the exception to the calling method. It's crucial to handle or declare checked exceptions to avoid compilation errors."
5. What is the Difference Between Checked and Unchecked Exceptions?
Why you might get asked this:
This question tests your understanding of the key differences between checked and unchecked exceptions and their implications for exception handling.
How to answer:
Explain that checked exceptions are checked at compile time, while unchecked exceptions are checked at runtime.
Mention that checked exceptions must be handled or declared, while unchecked exceptions do not require explicit handling.
Provide examples of each type, such as
IOException
for checked andNullPointerException
for unchecked.
Example answer:
"The main difference is that checked exceptions are checked at compile time, meaning the compiler enforces that you handle them using try-catch blocks or declare them using the throws clause. Unchecked exceptions, on the other hand, are checked at runtime and do not require explicit handling. For example, IOException
is a checked exception, while NullPointerException
is an unchecked exception."
6. How to Catch Multiple Exceptions?
Why you might get asked this:
This question assesses your knowledge of how to handle different types of exceptions within a single try block.
How to answer:
Explain that multiple catch blocks can be used to handle different exception types.
Mention that a single catch block can handle multiple exceptions using the
|
operator (Java 7 and later).Highlight the importance of ordering catch blocks from most specific to least specific.
Example answer:
"You can catch multiple exceptions by using multiple catch blocks, each handling a different exception type. In Java 7 and later, you can also use a single catch block to handle multiple exceptions using the |
operator. It's important to order your catch blocks from most specific to least specific to avoid compilation errors."
7. What is the Use of Finally Block?
Why you might get asked this:
This question tests your understanding of the purpose and usage of the finally block in exception handling.
How to answer:
Explain that the finally block executes regardless of whether an exception occurred or not.
Mention that it is typically used for resource cleanup, such as closing files or releasing network connections.
Highlight that the finally block ensures that critical cleanup code is always executed.
Example answer:
"The finally block is used to execute code that must run regardless of whether an exception occurred or not. It's typically used for resource cleanup, such as closing files, releasing network connections, or freeing up system resources. The finally block ensures that this critical cleanup code is always executed, even if an exception is thrown."
8. What is Exception Handling?
Why you might get asked this:
This question aims to assess your overall understanding of the concept and importance of exception handling in programming.
How to answer:
Define exception handling as a mechanism to deal with runtime errors and maintain the program's flow.
Explain that it involves identifying potential exceptions, catching them, and taking appropriate actions.
Highlight the benefits of exception handling, such as preventing program crashes and providing a better user experience.
Example answer:
"Exception handling is a mechanism to deal with runtime errors in a program and maintain its normal flow. It involves identifying potential exceptions, catching them, and taking appropriate actions to recover from the error or gracefully terminate the program. Effective exception handling prevents program crashes and provides a better user experience by handling errors in a controlled manner."
9. Explain the try-catch block.
Why you might get asked this:
This question tests your knowledge of the fundamental structure used for handling exceptions in Java.
How to answer:
Explain that the try block contains the code that might throw an exception.
Describe that the catch block contains the code that handles the exception if it occurs.
Mention that multiple catch blocks can be used to handle different types of exceptions.
Example answer:
"The try-catch block is a fundamental structure for handling exceptions in Java. The try block contains the code that might throw an exception. If an exception occurs within the try block, the corresponding catch block is executed to handle the exception. Multiple catch blocks can be used to handle different types of exceptions, allowing for specific error handling strategies for each type."
10. What is the purpose of the throws keyword?
Why you might get asked this:
This question assesses your understanding of how to declare that a method might throw an exception, shifting the responsibility of handling it to the calling method.
How to answer:
Explain that the throws keyword is used in a method signature to declare that the method might throw a checked exception.
Mention that it indicates that the method does not handle the exception itself and passes the responsibility to the calling method.
Highlight that the calling method must then either handle the exception or declare it using throws as well.
Example answer:
"The throws keyword is used in a method signature to declare that the method might throw a checked exception. It indicates that the method does not handle the exception itself and passes the responsibility of handling it to the calling method. The calling method must then either handle the exception using a try-catch block or declare it using throws as well, propagating the exception further up the call stack."
11. What are custom exceptions?
Why you might get asked this:
This question tests your understanding of when and how to create your own exception types to handle specific error conditions.
How to answer:
Define custom exceptions as user-defined exceptions that extend the
Exception
class or its subclasses.Explain that they are used to handle specific error conditions that are not covered by built-in exceptions.
Mention that custom exceptions can provide more specific information about the error.
Example answer:
"Custom exceptions are user-defined exceptions that extend the Exception
class or its subclasses. They are used to handle specific error conditions that are not covered by built-in exceptions. Custom exceptions can provide more specific information about the error, making it easier to diagnose and handle in the application."
12. How do you create a custom exception in Java?
Why you might get asked this:
This question evaluates your practical skills in implementing custom exceptions to address specific error scenarios in your code.
How to answer:
Explain that you create a custom exception by extending the
Exception
class or one of its subclasses.Mention that you should provide constructors to set the exception message and any other relevant data.
Highlight that you can add custom fields and methods to provide additional information about the exception.
Example answer:
"To create a custom exception in Java, you extend the Exception
class or one of its subclasses, such as RuntimeException
. You should provide constructors to set the exception message and any other relevant data. You can also add custom fields and methods to provide additional information about the exception, making it more informative and easier to handle."
13. What is the difference between throw and throws?
Why you might get asked this:
This question tests your ability to distinguish between the two keywords used for exception handling in Java.
How to answer:
Explain that throw is used to explicitly throw an exception within a method.
Describe that throws is used in a method signature to declare that the method might throw an exception.
Highlight that throw is a statement, while throws is a clause in the method declaration.
Example answer:
"The keyword throw
is used to explicitly throw an exception within a method, indicating that an error condition has occurred. The keyword throws
is used in a method signature to declare that the method might throw one or more exceptions, shifting the responsibility of handling those exceptions to the calling method. throw
is a statement used to initiate an exception, while throws
is a clause in the method declaration."
14. Can a finally block be skipped?
Why you might get asked this:
This question assesses your understanding of the conditions under which a finally block might not execute.
How to answer:
Explain that a finally block is generally always executed, regardless of whether an exception occurs.
Mention that it can be skipped if the JVM crashes or if
System.exit()
is called.Highlight that it can also be skipped if there is an infinite loop or a deadlock.
Example answer:
"A finally block is generally always executed, regardless of whether an exception occurs in the try block. However, there are a few scenarios where the finally block can be skipped: if the JVM crashes, if System.exit()
is called, or if there is an infinite loop or a deadlock that prevents the finally block from being reached. These cases are rare but important to be aware of."
15. What happens if an exception is not caught?
Why you might get asked this:
This question tests your understanding of the consequences of not handling an exception and how it affects the program's execution.
How to answer:
Explain that if an exception is not caught, the program will terminate abruptly.
Mention that the JVM will print the exception's stack trace to the console.
Highlight that any code after the point where the exception was thrown will not be executed.
Example answer:
"If an exception is not caught, the program will terminate abruptly. The JVM will print the exception's stack trace to the console, which can be helpful for debugging but is not ideal for a production environment. Any code after the point where the exception was thrown will not be executed, potentially leaving resources unreleased and operations incomplete."
16. Explain exception propagation.
Why you might get asked this:
This question assesses your knowledge of how exceptions are passed up the call stack when they are not handled in the current method.
How to answer:
Explain that exception propagation is the process of an exception being passed up the call stack to the calling method.
Mention that this continues until the exception is caught or the top of the call stack is reached.
Highlight that if the exception reaches the top of the call stack without being caught, the program terminates.
Example answer:
"Exception propagation is the process of an exception being passed up the call stack to the calling method when it is not caught in the current method. This process continues until the exception is caught by a suitable catch block or until the top of the call stack is reached. If the exception reaches the top of the call stack without being caught, the program terminates, and the exception's stack trace is printed to the console."
17. What is the difference between Error and Exception?
Why you might get asked this:
This question tests your understanding of the distinction between errors and exceptions in Java and how they should be handled differently.
How to answer:
Explain that both Error and Exception are subclasses of Throwable.
Mention that Errors represent serious problems that are generally unrecoverable, while Exceptions represent conditions that a program might want to catch.
Highlight that Errors are typically not caught, while Exceptions are often handled using try-catch blocks.
Example answer:
"Both Error and Exception are subclasses of Throwable, but they represent different types of problems. Errors represent serious problems that are generally unrecoverable, such as OutOfMemoryError
or StackOverflowError
. Exceptions, on the other hand, represent conditions that a program might want to catch and handle, such as IOException
or NullPointerException
. Errors are typically not caught by applications, while Exceptions are often handled using try-catch blocks to prevent program termination."
18. Can you have an empty catch block? Is it good practice?
Why you might get asked this:
This question assesses your understanding of best practices in exception handling and the potential pitfalls of using empty catch blocks.
How to answer:
Explain that it is possible to have an empty catch block, but it is generally not good practice.
Mention that an empty catch block can hide errors and make debugging difficult.
Highlight that at a minimum, the catch block should log the exception or re-throw it.
Example answer:
"Yes, it is possible to have an empty catch block, but it is generally not good practice. An empty catch block can hide errors and make debugging difficult because it effectively ignores the exception without taking any action. At a minimum, the catch block should log the exception or re-throw it to ensure that the error is not silently ignored."
19. How does exception handling affect performance?
Why you might get asked this:
This question tests your awareness of the performance implications of using exception handling in your code.
How to answer:
Explain that throwing and catching exceptions can be expensive in terms of performance.
Mention that the JVM needs to create a stack trace when an exception is thrown, which can be time-consuming.
Highlight that using exceptions for normal control flow should be avoided.
Example answer:
"Exception handling can affect performance because throwing and catching exceptions can be expensive. The JVM needs to create a stack trace when an exception is thrown, which can be a time-consuming operation. Therefore, it's important to use exception handling judiciously and avoid using exceptions for normal control flow. Instead, exceptions should be reserved for handling truly exceptional situations."
20. What are some best practices for exception handling?
Why you might get asked this:
This question assesses your knowledge of recommended guidelines for writing robust and maintainable code using exception handling.
How to answer:
Mention specific exception types over general ones.
Explain throwing exceptions early when they occur.
Describe catching them late where they can be meaningfully handled.
Highlight always logging meaningful messages when catching an exception.
Explain using custom messages if standard ones don't fit your use case.
Example answer:
"Some best practices for exception handling include: using specific exception types instead of general ones, throwing exceptions early when they occur, catching them late where they can be meaningfully handled, always logging meaningful messages when catching an exception, and using custom messages if standard ones don't fit your use case. Following these practices helps maintain robustness and readability in code."
21. How do you log exceptions?
Why you might get asked this:
This question evaluates your understanding of how to properly record exceptions for debugging and monitoring purposes.
How to answer:
Explain that you can use a logging framework like Log4j or SLF4j to log exceptions.
Mention that you should log the exception message, stack trace, and any other relevant information.
Highlight that logging should be done in a way that allows for easy analysis and troubleshooting.
Example answer:
"To log exceptions, you can use a logging framework like Log4j or SLF4j. When logging an exception, you should include the exception message, the full stack trace, and any other relevant information that can help diagnose the issue. Logging should be done in a way that allows for easy analysis and troubleshooting, such as using appropriate log levels and structured logging formats."
22. What is the purpose of chained exceptions?
Why you might get asked this:
This question tests your knowledge of how to link exceptions together to provide a more complete context of the error.
How to answer:
Explain that chained exceptions allow you to associate one exception with another.
Mention that this is useful when an exception is caused by another exception.
Highlight that chained exceptions provide a more complete context of the error, making it easier to diagnose.
Example answer:
"Chained exceptions allow you to associate one exception with another, providing a more complete context of the error. This is useful when an exception is caused by another exception, such as when a database connection error causes a higher-level application error. By chaining the exceptions, you can preserve the original error information and make it easier to diagnose the root cause of the problem."
23. How do you re-throw an exception?
Why you might get asked this:
This question assesses your understanding of how to handle an exception in one context and then pass it up the call stack for further handling.
How to answer:
Explain that you can re-throw an exception by using the throw keyword in the catch block.
Mention that you can re-throw the original exception or wrap it in a new exception.
Highlight that re-throwing an exception allows you to handle it partially and then pass it up the call stack for further handling.
Example answer:
"You can re-throw an exception by using the throw
keyword in the catch block. You can re-throw the original exception or wrap it in a new exception, providing additional context or information. Re-throwing an exception allows you to handle it partially in one context and then pass it up the call stack for further handling or logging."
24. What is try-with-resources in Java?
Why you might get asked this:
This question tests your knowledge of a modern feature in Java that simplifies resource management and exception handling.
How to answer:
Explain that try-with-resources is a feature introduced in Java 7 that automatically closes resources after use.
Mention that it requires the resource to implement the AutoCloseable interface.
Highlight that it simplifies resource management and reduces the risk of resource leaks.
Example answer:
"Try-with-resources is a feature introduced in Java 7 that automatically closes resources after use, simplifying resource management and reducing the risk of resource leaks. It requires the resource to implement the AutoCloseable
interface, which includes a close()
method. When the try block completes, the close()
method is automatically called, ensuring that the resource is properly released."
25. Can you use multiple try-catch blocks?
Why you might get asked this:
This question assesses your understanding of how to structure exception handling logic with multiple potential error points.
How to answer:
Explain that you can use multiple try-catch blocks to handle different sections of code that might throw exceptions.
Mention that each try-catch block can handle different types of exceptions.
Highlight that this allows for more granular and specific error handling.
Example answer:
"Yes, you can use multiple try-catch blocks to handle different sections of code that might throw exceptions. Each try-catch block can handle different types of exceptions, allowing for more granular and specific error handling. This approach is useful when different parts of your code might throw different exceptions that require different handling strategies."
26. What are some common exceptions in Java?
Why you might get asked this:
This question tests your familiarity with the most frequently encountered exceptions in Java development.
How to answer:
Mention
NullPointerException
,IOException
,ArrayIndexOutOfBoundsException
,ClassNotFoundException
, andIllegalArgumentException
.Explain the common causes of each exception.
Highlight the importance of understanding these exceptions for effective debugging and error handling.
Example answer:
"Some common exceptions in Java include NullPointerException
, which occurs when you try to access a member of a null object; IOException
, which occurs during input or output operations; ArrayIndexOutOfBoundsException
, which occurs when you try to access an array element with an invalid index; ClassNotFoundException
, which occurs when the JVM tries to load a class that is not found; and IllegalArgumentException
, which occurs when a method receives an argument that is not valid. Understanding these exceptions is crucial for effective debugging and error handling."
27. How do you handle exceptions in multi-threaded environments?
Why you might get asked this:
This question assesses your understanding of the challenges and best practices for handling exceptions in concurrent programs.
How to answer:
Explain that exceptions in one thread should not affect other threads.
Mention that each thread should have its own try-catch blocks to handle exceptions.
Highlight that you should use appropriate synchronization mechanisms to prevent race conditions and data corruption.
Example answer:
"In multi-threaded environments, it's important to ensure that exceptions in one thread do not affect other threads. Each thread should have its own try-catch blocks to handle exceptions independently. Additionally, you should use appropriate synchronization mechanisms, such as locks or atomic variables, to prevent race conditions and data corruption when handling exceptions in shared resources."
28. Explain the concept of exception masking.
Why you might get asked this:
This question tests your knowledge of a potential pitfall in exception handling where important exceptions are hidden or lost.
How to answer:
Explain that exception masking occurs when an exception is caught and a new exception is thrown, but the original exception is lost.
Mention that this can make it difficult to diagnose the root cause of the problem.
Highlight that you should use chained exceptions or logging to preserve the original exception information.
Example answer:
"Exception masking occurs when an exception is caught, and a new exception is thrown, but the original exception is lost. This can make it difficult to diagnose the root cause of the problem because the original error information is not preserved. To avoid exception masking, you should use chained exceptions or logging to preserve the original exception information and provide a more complete context of the error."
29. What are the advantages of using exceptions?
Why you might get asked this:
This question assesses your understanding of the benefits of using exceptions for error handling compared to other approaches.
How to answer:
Explain that exceptions provide a structured and standardized way to handle errors.
Mention that exceptions allow you to separate error-handling code from normal code.
Highlight that exceptions can improve code readability and maintainability.
Example answer:
"The advantages of using exceptions include providing a structured and standardized way to handle errors, allowing you to separate error-handling code from normal code, and improving code readability and maintainability. Exceptions also allow you to propagate errors up the call stack to a suitable handler, ensuring that errors are not silently ignored."
30. How do you design an exception handling strategy for a large application?
Why you might get asked this:
This question tests your ability to think strategically about exception handling in complex systems.
How to answer:
Explain that you should identify potential exceptions and categorize them based on severity.
Mention that you should define a consistent logging strategy for all exceptions.
Highlight that you should use custom exceptions to handle application-specific error conditions.
Explain that you should centralize exception handling logic where appropriate to avoid code duplication.
Example answer:
"Designing an exception handling strategy for a large application involves several key steps. First, you should identify potential exceptions and categorize them based on severity. Then, you should define a consistent logging strategy for all exceptions, ensuring that all relevant information is captured. You should also use custom exceptions to handle application-specific error conditions. Finally, you should centralize exception handling logic where appropriate to avoid code duplication and ensure consistency across the application."
Other Tips to Prepare for a Exception Handling Interview
Review Core Concepts: Ensure you have a solid understanding of the basics, such as what exceptions are, the different types of exceptions, and the try-catch-finally block.
Practice Coding: Write code that throws and catches exceptions. Experiment with different scenarios and error conditions.
Study Common Exceptions: Familiarize yourself with common exceptions like
NullPointerException
,IOException
, andIllegalArgumentException
.Understand Best Practices: Learn and apply best practices for exception handling, such as using specific exception types and logging exceptions properly.
Mock Interviews: Practice answering exception handling interview questions with a friend or mentor.
Ace Your Interview with Verve AI
Need a boost for your upcoming interviews? Sign up for Verve AI—your all-in-one AI-powered interview partner. With tools like the Interview Copilot, AI Resume Builder, and AI Mock Interview, Verve AI gives you real-time guidance, company-specific scenarios, and smart feedback tailored to your goals. Join thousands of candidates who've used Verve AI to land their dream roles with confidence and ease. 👉 Learn more and get started for free at https://vervecopilot.com/.
FAQ
Q: What is the most important thing to remember about exception handling?
A: The most important thing is to handle exceptions in a way that prevents the program from crashing and provides a graceful user experience. This involves catching exceptions, logging them, and taking appropriate actions to recover from the error or terminate the program gracefully.
Q: How can I improve my exception handling skills?
A: You can improve your exception handling skills by studying the core concepts, practicing coding, and learning from experienced developers. Pay attention to best practices and try to anticipate potential error conditions in your code.
Q: Is it always necessary to catch exceptions?
A: No, it is not always necessary to catch exceptions. In some cases, it might be appropriate to allow an exception to propagate up the call stack to a higher-level handler. However, you should always ensure that exceptions are handled somewhere in the application to prevent program crashes.
By preparing thoroughly and practicing your answers, you can confidently tackle exception handling interview questions and demonstrate your expertise to potential employers. Good luck!
Introduction to Exception Handling Interview Questions
Preparing for interviews, especially those involving technical topics like exception handling, can be daunting. However, mastering common interview questions can significantly boost your confidence and performance. This guide covers 30 of the most frequently asked exception handling interview questions, providing you with insights, strategies, and sample answers to help you ace your next interview.
What are Exception Handling Interview Questions?
Exception handling interview questions are designed to evaluate your understanding of how to manage errors and unexpected events in a program. These questions assess your knowledge of exception types, handling mechanisms, and best practices for writing robust and reliable code. Interviewers use these questions to gauge your ability to prevent application crashes, ensure graceful error recovery, and maintain a positive user experience even when things go wrong.
Why Do Interviewers Ask Exception Handling Questions?
Interviewers ask exception handling questions to determine if you can write stable, maintainable, and user-friendly applications. They want to see if you understand:
Error Management: How well you can anticipate and handle potential errors.
Code Robustness: Your ability to write code that doesn't crash unexpectedly.
Debugging Skills: How effectively you can identify and fix issues.
Best Practices: Whether you follow recommended guidelines for exception handling.
Problem-Solving: Your approach to resolving unexpected issues in a systematic way.
By evaluating these aspects, interviewers can assess whether you have the skills necessary to build resilient software that can withstand real-world conditions.
Preview of 30 Exception Handling Interview Questions
What is an Exception in Java?
What is the Superclass of All Exceptions in Java?
What are the Types of Exceptions in Java?
How to Handle Checked Exceptions?
What is the Difference Between Checked and Unchecked Exceptions?
How to Catch Multiple Exceptions?
What is the Use of Finally Block?
What is Exception Handling?
Explain the try-catch block.
What is the purpose of the throws keyword?
What are custom exceptions?
How do you create a custom exception in Java?
What is the difference between throw and throws?
Can a finally block be skipped?
What happens if an exception is not caught?
Explain exception propagation.
What is the difference between Error and Exception?
Can you have an empty catch block? Is it good practice?
How does exception handling affect performance?
What are some best practices for exception handling?
How do you log exceptions?
What is the purpose of chained exceptions?
How do you re-throw an exception?
What is try-with-resources in Java?
Can you use multiple try-catch blocks?
What are some common exceptions in Java?
How do you handle exceptions in multi-threaded environments?
Explain the concept of exception masking.
What are the advantages of using exceptions?
How do you design an exception handling strategy for a large application?
30 Exception Handling Interview Questions
1. What is an Exception in Java?
Why you might get asked this:
Interviewers ask this question to gauge your basic understanding of what exceptions are and their role in program execution. It helps determine if you can define the concept accurately.
How to answer:
Define an exception as an event that disrupts the normal flow of the program.
Explain that exceptions occur during runtime due to various factors.
Mention examples such as network errors, database connection issues, or logical errors.
Example answer:
"An exception in Java is an event that occurs during the execution of a program, disrupting its normal flow. It can be caused by various factors such as network errors, database connection issues, or logical errors in the code."
2. What is the Superclass of All Exceptions in Java?
Why you might get asked this:
This question tests your knowledge of the Java exception hierarchy and the root class from which all exceptions are derived.
How to answer:
Identify
Throwable
as the superclass for all exceptions and errors.Explain that
Exception
is a subclass ofThrowable
and serves as the superclass for most exceptions.Mention that
Error
is another subclass ofThrowable
, representing serious problems that are generally unrecoverable.
Example answer:
"The superclass of all exceptions in Java is Throwable
. Exception
is a subclass of Throwable
and serves as the superclass for most exceptions that a program might want to catch. Error
is another subclass of Throwable
, representing serious problems like OutOfMemoryError
that are generally unrecoverable."
3. What are the Types of Exceptions in Java?
Why you might get asked this:
This question assesses your understanding of the different categories of exceptions in Java and how they are classified.
How to answer:
Describe the two main types: checked and unchecked exceptions.
Explain that checked exceptions are anticipated and must be handled at compile time.
Explain that unchecked exceptions occur due to programming errors and do not require explicit handling.
Mention custom exceptions as user-defined exceptions.
Example answer:
"There are two main types of exceptions in Java: checked and unchecked. Checked exceptions, like IOException
, must be handled at compile time, typically using a try-catch block or a throws clause. Unchecked exceptions, like NullPointerException
, occur due to programming errors and do not require explicit handling. Additionally, there are custom exceptions, which are user-defined exceptions created by extending the Exception
class."
4. How to Handle Checked Exceptions?
Why you might get asked this:
This question evaluates your practical knowledge of how to deal with exceptions that the compiler forces you to handle.
How to answer:
Explain that checked exceptions can be handled using a try-catch block.
Describe the alternative of declaring the exception with a throws clause in the method signature.
Highlight the importance of handling or declaring checked exceptions to avoid compilation errors.
Example answer:
"Checked exceptions can be handled in two primary ways: using a try-catch block to catch and handle the exception, or by declaring the exception with a throws clause in the method signature, which passes the responsibility of handling the exception to the calling method. It's crucial to handle or declare checked exceptions to avoid compilation errors."
5. What is the Difference Between Checked and Unchecked Exceptions?
Why you might get asked this:
This question tests your understanding of the key differences between checked and unchecked exceptions and their implications for exception handling.
How to answer:
Explain that checked exceptions are checked at compile time, while unchecked exceptions are checked at runtime.
Mention that checked exceptions must be handled or declared, while unchecked exceptions do not require explicit handling.
Provide examples of each type, such as
IOException
for checked andNullPointerException
for unchecked.
Example answer:
"The main difference is that checked exceptions are checked at compile time, meaning the compiler enforces that you handle them using try-catch blocks or declare them using the throws clause. Unchecked exceptions, on the other hand, are checked at runtime and do not require explicit handling. For example, IOException
is a checked exception, while NullPointerException
is an unchecked exception."
6. How to Catch Multiple Exceptions?
Why you might get asked this:
This question assesses your knowledge of how to handle different types of exceptions within a single try block.
How to answer:
Explain that multiple catch blocks can be used to handle different exception types.
Mention that a single catch block can handle multiple exceptions using the
|
operator (Java 7 and later).Highlight the importance of ordering catch blocks from most specific to least specific.
Example answer:
"You can catch multiple exceptions by using multiple catch blocks, each handling a different exception type. In Java 7 and later, you can also use a single catch block to handle multiple exceptions using the |
operator. It's important to order your catch blocks from most specific to least specific to avoid compilation errors."
7. What is the Use of Finally Block?
Why you might get asked this:
This question tests your understanding of the purpose and usage of the finally block in exception handling.
How to answer:
Explain that the finally block executes regardless of whether an exception occurred or not.
Mention that it is typically used for resource cleanup, such as closing files or releasing network connections.
Highlight that the finally block ensures that critical cleanup code is always executed.
Example answer:
"The finally block is used to execute code that must run regardless of whether an exception occurred or not. It's typically used for resource cleanup, such as closing files, releasing network connections, or freeing up system resources. The finally block ensures that this critical cleanup code is always executed, even if an exception is thrown."
8. What is Exception Handling?
Why you might get asked this:
This question aims to assess your overall understanding of the concept and importance of exception handling in programming.
How to answer:
Define exception handling as a mechanism to deal with runtime errors and maintain the program's flow.
Explain that it involves identifying potential exceptions, catching them, and taking appropriate actions.
Highlight the benefits of exception handling, such as preventing program crashes and providing a better user experience.
Example answer:
"Exception handling is a mechanism to deal with runtime errors in a program and maintain its normal flow. It involves identifying potential exceptions, catching them, and taking appropriate actions to recover from the error or gracefully terminate the program. Effective exception handling prevents program crashes and provides a better user experience by handling errors in a controlled manner."
9. Explain the try-catch block.
Why you might get asked this:
This question tests your knowledge of the fundamental structure used for handling exceptions in Java.
How to answer:
Explain that the try block contains the code that might throw an exception.
Describe that the catch block contains the code that handles the exception if it occurs.
Mention that multiple catch blocks can be used to handle different types of exceptions.
Example answer:
"The try-catch block is a fundamental structure for handling exceptions in Java. The try block contains the code that might throw an exception. If an exception occurs within the try block, the corresponding catch block is executed to handle the exception. Multiple catch blocks can be used to handle different types of exceptions, allowing for specific error handling strategies for each type."
10. What is the purpose of the throws keyword?
Why you might get asked this:
This question assesses your understanding of how to declare that a method might throw an exception, shifting the responsibility of handling it to the calling method.
How to answer:
Explain that the throws keyword is used in a method signature to declare that the method might throw a checked exception.
Mention that it indicates that the method does not handle the exception itself and passes the responsibility to the calling method.
Highlight that the calling method must then either handle the exception or declare it using throws as well.
Example answer:
"The throws keyword is used in a method signature to declare that the method might throw a checked exception. It indicates that the method does not handle the exception itself and passes the responsibility of handling it to the calling method. The calling method must then either handle the exception using a try-catch block or declare it using throws as well, propagating the exception further up the call stack."
11. What are custom exceptions?
Why you might get asked this:
This question tests your understanding of when and how to create your own exception types to handle specific error conditions.
How to answer:
Define custom exceptions as user-defined exceptions that extend the
Exception
class or its subclasses.Explain that they are used to handle specific error conditions that are not covered by built-in exceptions.
Mention that custom exceptions can provide more specific information about the error.
Example answer:
"Custom exceptions are user-defined exceptions that extend the Exception
class or its subclasses. They are used to handle specific error conditions that are not covered by built-in exceptions. Custom exceptions can provide more specific information about the error, making it easier to diagnose and handle in the application."
12. How do you create a custom exception in Java?
Why you might get asked this:
This question evaluates your practical skills in implementing custom exceptions to address specific error scenarios in your code.
How to answer:
Explain that you create a custom exception by extending the
Exception
class or one of its subclasses.Mention that you should provide constructors to set the exception message and any other relevant data.
Highlight that you can add custom fields and methods to provide additional information about the exception.
Example answer:
"To create a custom exception in Java, you extend the Exception
class or one of its subclasses, such as RuntimeException
. You should provide constructors to set the exception message and any other relevant data. You can also add custom fields and methods to provide additional information about the exception, making it more informative and easier to handle."
13. What is the difference between throw and throws?
Why you might get asked this:
This question tests your ability to distinguish between the two keywords used for exception handling in Java.
How to answer:
Explain that throw is used to explicitly throw an exception within a method.
Describe that throws is used in a method signature to declare that the method might throw an exception.
Highlight that throw is a statement, while throws is a clause in the method declaration.
Example answer:
"The keyword throw
is used to explicitly throw an exception within a method, indicating that an error condition has occurred. The keyword throws
is used in a method signature to declare that the method might throw one or more exceptions, shifting the responsibility of handling those exceptions to the calling method. throw
is a statement used to initiate an exception, while throws
is a clause in the method declaration."
14. Can a finally block be skipped?
Why you might get asked this:
This question assesses your understanding of the conditions under which a finally block might not execute.
How to answer:
Explain that a finally block is generally always executed, regardless of whether an exception occurs.
Mention that it can be skipped if the JVM crashes or if
System.exit()
is called.Highlight that it can also be skipped if there is an infinite loop or a deadlock.
Example answer:
"A finally block is generally always executed, regardless of whether an exception occurs in the try block. However, there are a few scenarios where the finally block can be skipped: if the JVM crashes, if System.exit()
is called, or if there is an infinite loop or a deadlock that prevents the finally block from being reached. These cases are rare but important to be aware of."
15. What happens if an exception is not caught?
Why you might get asked this:
This question tests your understanding of the consequences of not handling an exception and how it affects the program's execution.
How to answer:
Explain that if an exception is not caught, the program will terminate abruptly.
Mention that the JVM will print the exception's stack trace to the console.
Highlight that any code after the point where the exception was thrown will not be executed.
Example answer:
"If an exception is not caught, the program will terminate abruptly. The JVM will print the exception's stack trace to the console, which can be helpful for debugging but is not ideal for a production environment. Any code after the point where the exception was thrown will not be executed, potentially leaving resources unreleased and operations incomplete."
16. Explain exception propagation.
Why you might get asked this:
This question assesses your knowledge of how exceptions are passed up the call stack when they are not handled in the current method.
How to answer:
Explain that exception propagation is the process of an exception being passed up the call stack to the calling method.
Mention that this continues until the exception is caught or the top of the call stack is reached.
Highlight that if the exception reaches the top of the call stack without being caught, the program terminates.
Example answer:
"Exception propagation is the process of an exception being passed up the call stack to the calling method when it is not caught in the current method. This process continues until the exception is caught by a suitable catch block or until the top of the call stack is reached. If the exception reaches the top of the call stack without being caught, the program terminates, and the exception's stack trace is printed to the console."
17. What is the difference between Error and Exception?
Why you might get asked this:
This question tests your understanding of the distinction between errors and exceptions in Java and how they should be handled differently.
How to answer:
Explain that both Error and Exception are subclasses of Throwable.
Mention that Errors represent serious problems that are generally unrecoverable, while Exceptions represent conditions that a program might want to catch.
Highlight that Errors are typically not caught, while Exceptions are often handled using try-catch blocks.
Example answer:
"Both Error and Exception are subclasses of Throwable, but they represent different types of problems. Errors represent serious problems that are generally unrecoverable, such as OutOfMemoryError
or StackOverflowError
. Exceptions, on the other hand, represent conditions that a program might want to catch and handle, such as IOException
or NullPointerException
. Errors are typically not caught by applications, while Exceptions are often handled using try-catch blocks to prevent program termination."
18. Can you have an empty catch block? Is it good practice?
Why you might get asked this:
This question assesses your understanding of best practices in exception handling and the potential pitfalls of using empty catch blocks.
How to answer:
Explain that it is possible to have an empty catch block, but it is generally not good practice.
Mention that an empty catch block can hide errors and make debugging difficult.
Highlight that at a minimum, the catch block should log the exception or re-throw it.
Example answer:
"Yes, it is possible to have an empty catch block, but it is generally not good practice. An empty catch block can hide errors and make debugging difficult because it effectively ignores the exception without taking any action. At a minimum, the catch block should log the exception or re-throw it to ensure that the error is not silently ignored."
19. How does exception handling affect performance?
Why you might get asked this:
This question tests your awareness of the performance implications of using exception handling in your code.
How to answer:
Explain that throwing and catching exceptions can be expensive in terms of performance.
Mention that the JVM needs to create a stack trace when an exception is thrown, which can be time-consuming.
Highlight that using exceptions for normal control flow should be avoided.
Example answer:
"Exception handling can affect performance because throwing and catching exceptions can be expensive. The JVM needs to create a stack trace when an exception is thrown, which can be a time-consuming operation. Therefore, it's important to use exception handling judiciously and avoid using exceptions for normal control flow. Instead, exceptions should be reserved for handling truly exceptional situations."
20. What are some best practices for exception handling?
Why you might get asked this:
This question assesses your knowledge of recommended guidelines for writing robust and maintainable code using exception handling.
How to answer:
Mention specific exception types over general ones.
Explain throwing exceptions early when they occur.
Describe catching them late where they can be meaningfully handled.
Highlight always logging meaningful messages when catching an exception.
Explain using custom messages if standard ones don't fit your use case.
Example answer:
"Some best practices for exception handling include: using specific exception types instead of general ones, throwing exceptions early when they occur, catching them late where they can be meaningfully handled, always logging meaningful messages when catching an exception, and using custom messages if standard ones don't fit your use case. Following these practices helps maintain robustness and readability in code."
21. How do you log exceptions?
Why you might get asked this:
This question evaluates your understanding of how to properly record exceptions for debugging and monitoring purposes.
How to answer:
Explain that you can use a logging framework like Log4j or SLF4j to log exceptions.
Mention that you should log the exception message, stack trace, and any other relevant information.
Highlight that logging should be done in a way that allows for easy analysis and troubleshooting.
Example answer:
"To log exceptions, you can use a logging framework like Log4j or SLF4j. When logging an exception, you should include the exception message, the full stack trace, and any other relevant information that can help diagnose the issue. Logging should be done in a way that allows for easy analysis and troubleshooting, such as using appropriate log levels and structured logging formats."
22. What is the purpose of chained exceptions?
Why you might get asked this:
This question tests your knowledge of how to link exceptions together to provide a more complete context of the error.
How to answer:
Explain that chained exceptions allow you to associate one exception with another.
Mention that this is useful when an exception is caused by another exception.
Highlight that chained exceptions provide a more complete context of the error, making it easier to diagnose.
Example answer:
"Chained exceptions allow you to associate one exception with another, providing a more complete context of the error. This is useful when an exception is caused by another exception, such as when a database connection error causes a higher-level application error. By chaining the exceptions, you can preserve the original error information and make it easier to diagnose the root cause of the problem."
23. How do you re-throw an exception?
Why you might get asked this:
This question assesses your understanding of how to handle an exception in one context and then pass it up the call stack for further handling.
How to answer:
Explain that you can re-throw an exception by using the throw keyword in the catch block.
Mention that you can re-throw the original exception or wrap it in a new exception.
Highlight that re-throwing an exception allows you to handle it partially and then pass it up the call stack for further handling.
Example answer:
"You can re-throw an exception by using the throw
keyword in the catch block. You can re-throw the original exception or wrap it in a new exception, providing additional context or information. Re-throwing an exception allows you to handle it partially in one context and then pass it up the call stack for further handling or logging."
24. What is try-with-resources in Java?
Why you might get asked this:
This question tests your knowledge of a modern feature in Java that simplifies resource management and exception handling.
How to answer:
Explain that try-with-resources is a feature introduced in Java 7 that automatically closes resources after use.
Mention that it requires the resource to implement the AutoCloseable interface.
Highlight that it simplifies resource management and reduces the risk of resource leaks.
Example answer:
"Try-with-resources is a feature introduced in Java 7 that automatically closes resources after use, simplifying resource management and reducing the risk of resource leaks. It requires the resource to implement the AutoCloseable
interface, which includes a close()
method. When the try block completes, the close()
method is automatically called, ensuring that the resource is properly released."
25. Can you use multiple try-catch blocks?
Why you might get asked this:
This question assesses your understanding of how to structure exception handling logic with multiple potential error points.
How to answer:
Explain that you can use multiple try-catch blocks to handle different sections of code that might throw exceptions.
Mention that each try-catch block can handle different types of exceptions.
Highlight that this allows for more granular and specific error handling.
Example answer:
"Yes, you can use multiple try-catch blocks to handle different sections of code that might throw exceptions. Each try-catch block can handle different types of exceptions, allowing for more granular and specific error handling. This approach is useful when different parts of your code might throw different exceptions that require different handling strategies."
26. What are some common exceptions in Java?
Why you might get asked this:
This question tests your familiarity with the most frequently encountered exceptions in Java development.
How to answer:
Mention
NullPointerException
,IOException
,ArrayIndexOutOfBoundsException
,ClassNotFoundException
, andIllegalArgumentException
.Explain the common causes of each exception.
Highlight the importance of understanding these exceptions for effective debugging and error handling.
Example answer:
"Some common exceptions in Java include NullPointerException
, which occurs when you try to access a member of a null object; IOException
, which occurs during input or output operations; ArrayIndexOutOfBoundsException
, which occurs when you try to access an array element with an invalid index; ClassNotFoundException
, which occurs when the JVM tries to load a class that is not found; and IllegalArgumentException
, which occurs when a method receives an argument that is not valid. Understanding these exceptions is crucial for effective debugging and error handling."
27. How do you handle exceptions in multi-threaded environments?
Why you might get asked this:
This question assesses your understanding of the challenges and best practices for handling exceptions in concurrent programs.
How to answer:
Explain that exceptions in one thread should not affect other threads.
Mention that each thread should have its own try-catch blocks to handle exceptions.
Highlight that you should use appropriate synchronization mechanisms to prevent race conditions and data corruption.
Example answer:
"In multi-threaded environments, it's important to ensure that exceptions in one thread do not affect other threads. Each thread should have its own try-catch blocks to handle exceptions independently. Additionally, you should use appropriate synchronization mechanisms, such as locks or atomic variables, to prevent race conditions and data corruption when handling exceptions in shared resources."
28. Explain the concept of exception masking.
Why you might get asked this:
This question tests your knowledge of a potential pitfall in exception handling where important exceptions are hidden or lost.
How to answer:
Explain that exception masking occurs when an exception is caught and a new exception is thrown, but the original exception is lost.
Mention that this can make it difficult to diagnose the root cause of the problem.
Highlight that you should use chained exceptions or logging to preserve the original exception information.
Example answer:
"Exception masking occurs when an exception is caught, and a new exception is thrown, but the original exception is lost. This can make it difficult to diagnose the root cause of the problem because the original error information is not preserved. To avoid exception masking, you should use chained exceptions or logging to preserve the original exception information and provide a more complete context of the error."
29. What are the advantages of using exceptions?
Why you might get asked this:
This question assesses your understanding of the benefits of using exceptions for error handling compared to other approaches.
How to answer:
Explain that exceptions provide a structured and standardized way to handle errors.
Mention that exceptions allow you to separate error-handling code from normal code.
Highlight that exceptions can improve code readability and maintainability.
Example answer:
"The advantages of using exceptions include providing a structured and standardized way to handle errors, allowing you to separate error-handling code from normal code, and improving code readability and maintainability. Exceptions also allow you to propagate errors up the call stack to a suitable handler, ensuring that errors are not silently ignored."
30. How do you design an exception handling strategy for a large application?
Why you might get asked this:
This question tests your ability to think strategically about exception handling in complex systems.
How to answer:
Explain that you should identify potential exceptions and categorize them based on severity.
Mention that you should define a consistent logging strategy for all exceptions.
Highlight that you should use custom exceptions to handle application-specific error conditions.
Explain that you should centralize exception handling logic where appropriate to avoid code duplication.
Example answer:
"Designing an exception handling strategy for a large application involves several key steps. First, you should identify potential exceptions and categorize them based on severity. Then, you should define a consistent logging strategy for all exceptions, ensuring that all relevant information is captured. You should also use custom exceptions to handle application-specific error conditions. Finally, you should centralize exception handling logic where appropriate to avoid code duplication and ensure consistency across the application."
Other Tips to Prepare for a Exception Handling Interview
Review Core Concepts: Ensure you have a solid understanding of the basics, such as what exceptions are, the different types of exceptions, and the try-catch-finally block.
Practice Coding: Write code that throws and catches exceptions. Experiment with different scenarios and error conditions.
Study Common Exceptions: Familiarize yourself with common exceptions like
NullPointerException
,IOException
, andIllegalArgumentException
.Understand Best Practices: Learn and apply best practices for exception handling, such as using specific exception types and logging exceptions properly.
Mock Interviews: Practice answering exception handling interview questions with a friend or mentor.
Ace Your Interview with Verve AI
Need a boost for your upcoming interviews? Sign up for Verve AI—your all-in-one AI-powered interview partner. With tools like the Interview Copilot, AI Resume Builder, and AI Mock Interview, Verve AI gives you real-time guidance, company-specific scenarios, and smart feedback tailored to your goals. Join thousands of candidates who've used Verve AI to land their dream roles with confidence and ease. 👉 Learn more and get started for free at https://vervecopilot.com/.
FAQ
Q: What is the most important thing to remember about exception handling?
A: The most important thing is to handle exceptions in a way that prevents the program from crashing and provides a graceful user experience. This involves catching exceptions, logging them, and taking appropriate actions to recover from the error or terminate the program gracefully.
Q: How can I improve my exception handling skills?
A: You can improve your exception handling skills by studying the core concepts, practicing coding, and learning from experienced developers. Pay attention to best practices and try to anticipate potential error conditions in your code.
Q: Is it always necessary to catch exceptions?
A: No, it is not always necessary to catch exceptions. In some cases, it might be appropriate to allow an exception to propagate up the call stack to a higher-level handler. However, you should always ensure that exceptions are handled somewhere in the application to prevent program crashes.
By preparing thoroughly and practicing your answers, you can confidently tackle exception handling interview questions and demonstrate your expertise to potential employers. Good luck!
30 Most Common Linear Regression Interview Questions You Should Prepare For
MORE ARTICLES
MORE ARTICLES
MORE ARTICLES
Apr 11, 2025
Apr 11, 2025
Apr 11, 2025
30 Most Common mechanical fresher interview questions You Should Prepare For
30 Most Common mechanical fresher interview questions You Should Prepare For
Apr 7, 2025
Apr 7, 2025
Apr 7, 2025
30 Most Common WPF Interview Questions You Should Prepare For
30 Most Common WPF Interview Questions You Should Prepare For
Apr 11, 2025
Apr 11, 2025
Apr 11, 2025
30 Most Common Java Coding Interview Questions for 5 Years Experience
30 Most Common Java Coding Interview Questions for 5 Years Experience
Ace Your Next Interview with Real-Time AI Support
Ace Your Next Interview with Real-Time AI Support
Ace Your Next Interview with Real-Time AI Support
Get real-time support and personalized guidance to ace live interviews with confidence.
Get real-time support and personalized guidance to ace live interviews with confidence.
Get real-time support and personalized guidance to ace live interviews with confidence.
Become interview-ready in no time
Prep smarter and land your dream offers today!